No matter how smoothly your software application operates, a single exception caused by flawed coding can jeopardize its functionality, potentially impacting millions of users. This scenario underlines the importance of exception handling in any piece of code. This guide offers insightful recommendations, best practices, and effective strategies for handling exceptions in C#.
Delving Into The Concept of Exception Handling
At its core, an exception refers to an error that crops up during program execution, disrupting the usual progression or execution. Examples of exceptions are numerous and may range from invalid user input to unavailable resources like files and folders or an attempt to access non-existent indices in arrays.
Exception handling is a proactive strategy employed in coding to manage potential error conditions effectively, thereby allowing the program to continue running without interruption. By effectively understanding and implementing exception handling, developers can ensure that even in the face of unexpected events or conditions, the software performs reliably.
In this guide, you’ll gain a detailed understanding of:
- The core principles and concepts of exception handling in C#;
- Strategies for identifying, capturing, and managing exceptions effectively;
- The different types of exceptions, their cause, and how they impact your code;
- Best practices and key tips for leveraging C# exception handling to develop robust, reliable software
By the end of this guide, you’ll be equipped with the knowledge and insights to manage exceptions in your C# projects effectively, promoting error-free, efficient, and more user-friendly software applications.
Exception Handling in C#: Advanced Strategies and Best Practices
C# offers a multitude of methods for managing exceptions effectively, featuring elements such as try-catch blocks, multiple try-catch blocks, and user-defined custom exceptions. To develop a competent application, it’s important to adhere to certain best practices in exception handling.
Prioritizing Error Management Over Exception Throwing
Common or recurrent errors should be anticipated and strategically managed. A classic instance is the ‘divide by zero’ exception, which often emerges in division operations within the code.
int numerator = 100;
int denominator = 0;
try {
Console.WriteLine(numerator / denominator);
}
catch (DivideByZeroException) {
Console.WriteLine("Divide by zero exception occurred");
}
However, throwing exceptions for such frequently occurring errors isn’t ideal. Rather, preemptively checking if the denominator is zero and substituting it with a non-zero default value is a more efficient approach.
int numerator = 100;
int denominator = 0;
if (denominator == 0) {
denominator = 1; //setting a default value
}
Proactively Avoiding NullReferenceException
Another common exception is the “NullReferenceException,” which takes place when an object or variable is null. Instead of triggering an exception, the prudent approach is to examine whether the object or variable is null prior to carrying out any operations. C# enables this with the use of the ‘?’ symbol that automatically verifies if the object is null.
User user = null;
var name = user?.Name;
This way, by selectively applying these best practices, developers can build well-engineered applications that are robust and resilient against exceptions, thereby enhancing the user experience and the overall performance of the software.
Enhancing Exception Handling with Effective Logging Strategies
Logging plays an integral role in reinforcing exception handling across various programming languages. This process involves accurately recording the exception object, which encompasses valuable data such as stack trace details and the exception type – all crucial elements in identifying and troubleshooting the relevant issue. Another indispensable aspect is to communicate a coherent, grammatically correct error message to streamline the troubleshooting process.
Consider the following example:
try {
//division by zero operation
}
catch (DivideByZeroException exception) {
Logger.LogError(exception, "An exception has occurred due to division by zero.");
}
ClearInsights Exception Handling offers an efficient way to automate the logging process while ensuring that all exceptions are effectively tracked and assigned for resolution. This tool also sends email notifications to team members according to customized settings. A free account can be created on ClearInsights to explore these benefits.
Integration of ClearInsights Logging can be achieved with the following code:
builder.Logging.AddClearInsightsLogger(configuration => {
configuration.ApiKey = "{Your ApiKey}";
configuration.Secret = "{Your Environment Client Secret}";
configuration.ApplicationName = "{Your Application Name}";
});
Manual logging of each exception is not the most efficient strategy. Automating the process enhances the overall exception logging experience. Logging tools such as ClearInsights facilitate this by automatically recording a wealth of information including the exception message, product details, environment specifics, source, and stack trace of each log. ClearInsights also provides logging reports offering an overview of various log types such as Critical, Error, Warning, Informational, and Trace that offer different levels of logs.
In addition, ClearInsights allows users to filter and gain insights into frequently occurring exceptions in the system. As a best practice, the use of a comprehensive logging tool like ClearInsights is recommended. This ensures not only a seamless application logging experience, but the invaluable ability to trace anomalies back to their source.
Effective Stack Trace Preservation with Throw Statements
In the realm of exception handling with C#, ‘throw’ statements can prove instrumental. Some developers might find themselves using ‘throw ex’ when ‘throw’ would serve them better. The primary discrepancy lies in stack trace preservation – the ‘throw ex’ statement does not retain stack traces, making it challenging to pinpoint the error’s origin. On the other hand, the ‘throw’ statement diligently logs stack traces, advocating for better exception handling.
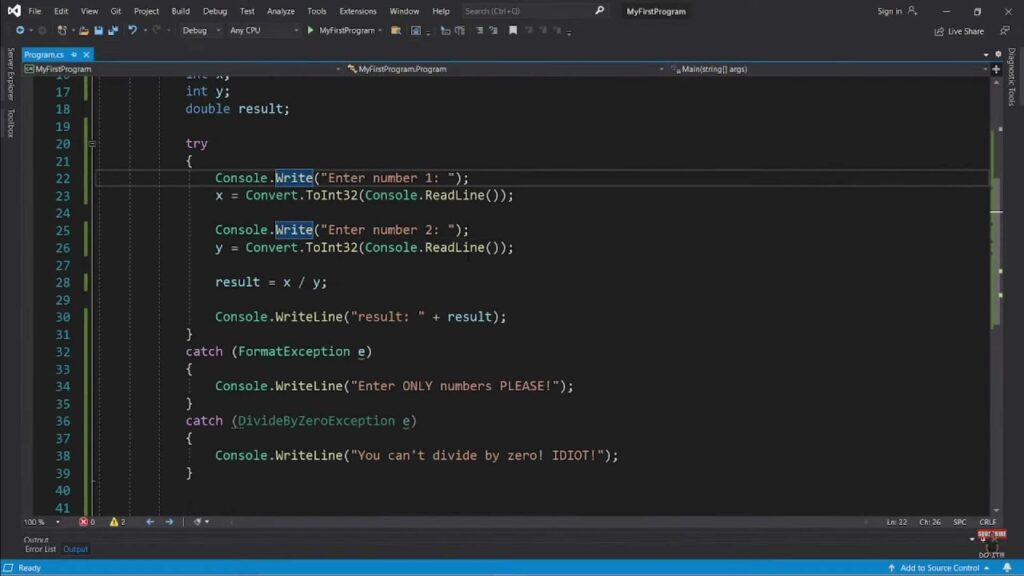
Consider the example below:
catch (Exception ex)
{
throw;
}
Empowering Exception Handling with Custom Exceptions
Custom exceptions, declared using the ‘Exception’ keyword, pave the way for a tailored approach to exception handling. See the following example:
public class MyCustomException: Exception {}
Custom exceptions allow you to define your own exception handling behavior and declare exceptions that frequently arise across your application classes. Including the following three constructors is recommended while defining custom exception classes:
Exception() - Sets default values.
Exception(String) - Allows you to pass a message.
Exception(String, Exception) - Sets an inner exception.
Example usage:
public class MyCustomException: Exception
{
public MyCustomException()
{
}
public MyCustomException(string message): base(message)
{
}
public MyCustomException(string message, Exception innerException): base(message, innerException)
{
}
}
Comprehensive logging should be integrated with every custom exception. This not only provides a robust exception-handling infrastructure but also eases the debugging process. Furthermore, using additional properties can offer insightful information about the exception, thereby facilitating a faster resolution.
By adopting these best practices, you can ensure that your applications are more resilient, debugging becomes less stressful, and your development workflow remains smooth. Discover the ultimate toolbox for developers with a visual studio subscription. Unleash your coding potential and boost productivity today!
Capitalizing on Predefined Exceptions: Streamlining Error Handling in C#
Skilled C# developers understand the power of leveraging predefined exceptions. While the fourth best practice advises creating custom exceptions to encapsulate application-specific error scenarios, this is not always necessary. In many cases, C# comes equipped with a host of predefined exceptions that can handle standard error cases efficiently. Thus, employing these can streamline your code and perhaps simplify debugging.
Here are some key predefined exceptions in C# and their typical use-cases:
- FileNotFoundException: This exception is thrown when a file that the program is trying to access cannot be found in the specified location. It’s helpful in file operations where the existence of the file is imperative for execution;
- DivideByZeroException: As the name suggests, this exception takes over when there is an attempt to divide a number by zero. This is common in arithmetic operations and can be a lifesaver when dealing with dynamic data prone to such errors;
- ArgumentNullException: This pops up when a null argument value gets used where it’s not allowed. Ensuring that no method argument or property setter is null before using them is a common practice in C# programming, and this exception acts as a safety net;
- InvalidOperationException: Triggered when an operation that is not legally permitted is attempted. This includes scenarios like manipulating an object in a state not suitable for the operation, such as trying to close an already closed network connection;
- IndexOutOfRangeException: This exception gets thrown when there’s an attempt to access an array’s element at an index beyond its valid range. This is common in operations involving arrays, lists, and other similar data structures.
Conclusion
In conclusion, using existing exceptions where applicable can make the code cleaner and easier to maintain. It also keeps the exception-handling mechanism aligned with the broader C# community’s conventions, which could prove beneficial for collaborative projects.
However, the use of custom exceptions for specific application-related error cases is still advised. This way, by combining predefined and custom exceptions, C# developers can sculpt a well-rounded exception-handling mechanism.