In the realm of programming, ensuring that your application runs smoothly and efficiently is of paramount importance. One aspect of achieving this is maintaining a robust system for tracking and managing log information. Python, a versatile and widely-used programming language, offers a powerful and flexible logging module that allows developers to easily capture and record essential information about their applications’ behavior.
Logging to a file is a fundamental component of any software development process, as it provides developers with insights into their code’s execution, helping them detect errors, monitor performance, and diagnose issues. However, for beginners, navigating Python’s logging module can initially seem like a complex task.
Fear not! In this beginner’s guide, we will demystify the art of logging in Python and focus specifically on the process of logging to a file. By the end of this article, you’ll be well-equipped to implement effective logging strategies in your Python applications, improving their reliability and maintainability. Let’s dive in and explore the world of Python logging to files.
Understanding Python’s Logging Module
Python’s logging module, an integral part of its standard library, is a sophisticated framework designed for recording and tracking events in Python applications. This module surpasses the basic functionality of print statements, offering a structured approach to gather insights about the operation and behavior of programs.
Significance in Software Development:
- Facilitates Debugging: By creating a detailed record of events, Python logging simplifies the process of identifying and resolving issues in the code. It provides a traceable path to the root cause of problems, enhancing the efficiency of the debugging process;
- Performance Monitoring: Logging data is invaluable for analyzing the application’s performance. It aids in identifying optimization needs and assists in scaling strategies;
- Insight into Usage Trends: Understanding how an application is used can be crucial for marketing and development strategies. Logging provides this insight by capturing usage patterns.
Basic Implementation:
To implement Python logging, the following steps are essential:
- Import the Logging Module;
- Begin by including the logging module in your Python script:
import logging
- Logging Levels;
- Python logging categorizes messages into different severity levels, each serving a unique purpose:
- Debug: For detailed diagnostic information;
- Info: General information about program execution;
- Warning: An indication of potential issues;
- Error: Highlighting errors that have occurred;
- Critical: For serious problems that might cause program termination.
Example usage:
logging.debug('Debug message')
logging.info('Info message')
logging.warning('Warning message')
logging.error('Error message')
logging.critical('Critical message')
Customizing Output:
The default format in Python logging, which includes the severity level, logger’s name, and the message, can be customized to include additional details such as timestamps and line numbers. This customization enhances the readability and utility of log messages.
Tips for Effective Logging:
- Appropriate Level Selection: Choose the right logging level to avoid cluttering the log files with unnecessary information;
- Consistent Format: Maintain a consistent format across log messages for easier analysis;
- Contextual Information: Include relevant contextual information in log messages to provide a clearer picture of the events;
- Regular Monitoring: Regularly review log files to preemptively identify and address potential issues.
Introduction to Python Logging Module
Python’s logging module is an extensive tool widely utilized across different skill levels, from beginners to enterprise applications. Its primary function is to facilitate structured and customizable logging for Python applications. This module offers a versatile approach to managing log records, including the ability to define multiple handlers for routing log messages.
Severity Levels in Python Logging
The Python logging module categorizes log messages into various severity levels. Each level represents the importance and type of the logged event, allowing for more effective debugging and monitoring.
Severity Levels and Descriptions:
- DEBUG (10): Provides in-depth insights for diagnosing issues;
- INFO (20): Confirms normal operation and expected outcomes;
- WARNING (30): Signals potential problems or upcoming challenges;
- ERROR (40): Indicates failures in specific tasks, signaling major issues;
- CRITICAL (50): Marks severe problems that might halt the program’s execution.
Functionalities and Methods of Python Logging
The Python logging module is rich in features, including a variety of constants, classes, and methods, enhancing its flexibility and usability.
Key Methods:
- Logger.info(msg): Logs an informational message;
- Logger.warning(msg): Logs a warning message;
- Logger.error(msg): Logs an error message;
- Logger.critical(msg): Logs a critical message;
- Logger.log(lvl, msg): Logs a message at a specific level;
- Logger.exception(msg): Logs an exception message;
- Logger.setLevel(lvl): Sets the threshold level for logging;
- Logger.addFilter(filt), Logger.removeFilter(filt): Manages filters;
- Logger.addHandler(hdlr), Logger.removeHandler(hdlr): Manages handlers.
- Logger.hasHandlers(): Checks for the existence of handlers.
Practical Example of Python Logging
To illustrate the use of the Python logging module, consider the following example code and its output:
import logging
- logging.debug(‘Debug message’);
- logging.info(‘Info message’);
- logging.warning(‘Warning message’);
- logging.error(‘Error message’);
- logging.critical(‘Critical message’).
Expected Output:
- WARNING:root: Warning message;
- ERROR:root: Error message;
- CRITICAL:root: Critical message.
This example demonstrates the default behavior of the logging module. By default, it logs messages at the WARNING level and above. Therefore, DEBUG and INFO messages are not displayed in the output.
Insights and Recommendations:
- Customization: Tailor the logging system using different handlers and levels to suit specific needs;
- Optimization: Use appropriate logging levels to avoid clutter and enhance clarity in log management;
- Best Practices: Regularly update and maintain logging configurations to keep pace with application changes.
Summary Table: Python Logging Levels
Level | Numeric Value |
---|---|
NOTSET | 0 |
DEBUG | 10 |
INFO | 20 |
WARNING | 30 |
ERROR | 40 |
CRITICAL | 50 |
Configuring and Formatting Log Records
Python’s logging system primarily records and stores event information in files. This functionality is enabled through the basicConfig(**kwargs) method from the logging module, which sets up the logging configurations.
Key arguments frequently used in Python’s logging setup include:
- level: Determines the severity threshold for logging;
- filename: Specifies the destination file for the log records;
- filemode: Dictates how the file is accessed. By default, files open in append mode (‘a’), allowing for the addition of new content;
- format: Defines the structure and content of the log messages.
The basicConfig() function also lets us specify filename, filemode, and format options. This way, we can tailor the log messages’ format and choose to either display them in the console or store them in a file. For instance, consider the following code:
import logging
logging.basicConfig(filename='msg.log', filemode='w', format='%(name)s - %(levelname)s - %(message)s')
logging.warning('This will log to a file')
This script logs messages to the msg.log file instead of the console. Opening the file in ‘write mode’ (‘w’) means it’s set to overwrite content with each execution. Additional arguments can be passed to basicConfig() to customize its behavior.
Regarding the output format, the log messages, which are strings, can be altered according to our requirements. These strings include several fundamental components of a LogRecord. For example:
import logging
logging.basicConfig(format='%(process)d-%(levelname)s-%(message)s')
logging.warning('This is a Warning Message')
This produces an output like 18472-WARNING-This is a Warning Message, showing how we can incorporate LogRecord attributes in various forms as the format argument in Python logging.
Enhanced Logging of Dynamic Data in Python Applications
In modern Python applications, it’s crucial to incorporate dynamic data within log files for effective debugging and monitoring. The Python logging module is adept at handling such requirements, but it requires a nuanced approach. Here’s a more detailed and insightful look into this process:
String Formatting with Dynamic Data:
- Overview: Python logging methods necessitate string arguments. To incorporate dynamic data, it’s advisable to format the string with this data before passing it to the logging function;
- Methodology: Utilize Python’s robust string formatting capabilities to embed variable data seamlessly into log messages;
- Tips:
- Ensure the clarity and readability of log messages;
- Avoid complex string concatenations; use formatted strings instead for simplicity and efficiency.
Utilizing Format Strings in Logging:
Technique: An alternative approach involves using a format string for the log message, appending variable data as an additional argument.
Example:
import logging
name = 'Harry Potter'
logging.error('%s raised an error', name)
Output Interpretation:
- The log output would be: root: Harry Potter raised an error;
- This method ensures that variable data, such as ‘Harry Potter’ in this case, is dynamically integrated into the log message.
Recording Complete Stack Traces in Python
For comprehensive debugging, recording complete stack traces in Python applications is invaluable. The Python logging module is equipped with functionalities to capture these details, especially in the event of exceptions. Here’s an expanded view:
Capturing Exception Details:
Method: Use the exc_info parameter within the logging function to record exception details.
Example:
import logging
a = 10
b = 0
try:
c = a / b
except Exception as e:
logging.error("Exception occurred", exc_info=True)
Output Analysis:
The output will show the exact line of the error (e.g., ZeroDivisionError: division by zero at c = a / b).
This detailed output is pivotal in tracing and fixing errors efficiently.
Using the exception() Method:
- Functionality: The exception() method in the Python logging module is tailored to capture and record exceptions in a detailed manner;
- Advantages:
- Automatically sets exc_info to True;
- Enhances the error logging with a prefixed ‘ERROR’ and provides the complete stack trace.
- Recommendations:
- Employ the exception() method in try-except blocks for optimal error logging;
- Tailor the logging level and message to suit the application’s needs and debugging practices.
Exploring Python’s Logging Module: Classes and Functions
Python’s logging module is a versatile toolkit for tracking events that occur during the execution of a program. It’s crucial for debugging and monitoring software behavior. This module provides several classes and functions to facilitate detailed logging.
Understanding the Root Logger
- Root Logger Fundamentals: This is the primary logger in Python’s logging system. It is used by default when logging functions like logging.debug() and logging.error() are invoked;
- Customization and Usage: One can customize logging by creating instances of the Logger class. This allows for more control over the logging process.
Key Classes in Python’s Logging Module
- Logger:
- Functionality: This class is used to call logging functions directly. By creating an instance of the Logger, tailored logging behavior can be achieved;
- Best Practices: Utilize Logger objects to manage different logging levels and to direct logs to specific destinations.
- LogRecord:
- Role: Automatically generates a record of each logging event;
- Details Captured: Includes vital information like the logger’s name, the function, line number, and the log message;
- Utility: Useful for in-depth analysis of events within an application.
- Handler:
- Purpose: Handles the dispatching of log records to various destinations;
- Types of Handlers: Includes FileHandler, StreamHandler, HTTPHandler, and SMTPHandler;
- Customization: Choose a handler based on the needs of the application, like logging to a file, streaming to a console, or sending over HTTP.
- Formatters:
- Function: Determines the format of the log output;
- Flexibility: Allows customization of log messages, making them more readable and informative;
- Default Format: The standard format for dates in log messages is %Y-%m-%d %H:%M:%S.
Leveraging getLogger for Consistent Logging
- getLogger Method: Used to create or retrieve Logger objects;
- Name-based Retrieval: If getLogger() is called multiple times with the same name, it returns a reference to the same Logger object;
- Consistency: This ensures consistency in logging across different parts of an application.
Tips for Effective Logging in Python
- Define Clear Logging Levels: Use different levels like DEBUG, INFO, WARNING, ERROR, and CRITICAL to categorize log messages;
- Use Formatters Wisely: Customize formatters to include information like timestamps, file names, and line numbers for more informative logs;
- Regular Review: Periodically review log files to monitor application health and identify potential issues early.
Utilizing Handlers for Effective Logging Management
Handlers in logging frameworks play a crucial role in managing and directing log messages to various destinations. These destinations can include different formats like emails, files, or standard console output. This flexibility allows for a streamlined approach to logging, ensuring that important information is captured and stored appropriately.
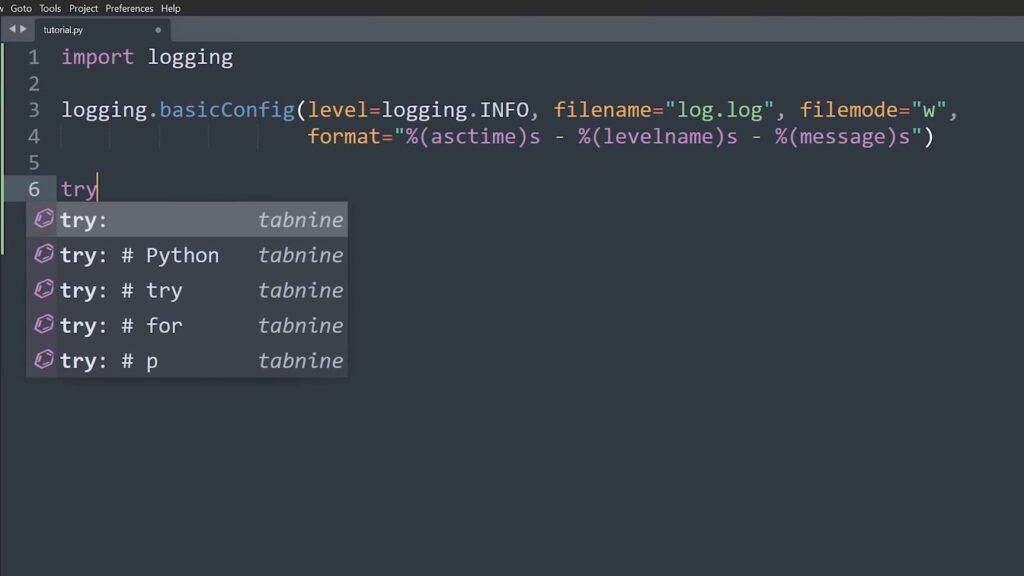
Creating and Configuring Handlers:
To effectively utilize handlers, one must first understand the process of creating and configuring them within a logging setup. This involves a few critical steps:
- Initialization of Logger:
- Begin by creating a unique logger object. This object acts as the starting point for all logging activities;
- Example: logger_obj = logging.getLogger(__name__);
- Setting Up Handlers:
- Establish different handlers for various logging outputs. For instance, StreamHandler for console output and FileHandler for writing logs to a file;
- Assign specific logging levels to each handler, like WARNING for StreamHandler and ERROR for FileHandler.
- Formatting Log Messages:
- Customize the format of log messages using Formatter. This adds clarity and structure to the logs;
- Different formats can be applied to different handlers to suit the needs of the output medium.
- Integrating Handlers with Logger:
- Attach the configured handlers to the logger object. This links the logger with the handlers, enabling log messages to be routed correctly.
Example of Handler Configuration in Python:
import logging
# Initialize the logger
logger_obj = logging.getLogger(__name__)
# Create and configure handlers
w_handler = logging.StreamHandler()
e_handler = logging.FileHandler('file.log')
w_handler.setLevel(logging.WARNING)
e_handler.setLevel(logging.ERROR)
# Format the log messages
c_format = logging.Formatter('%(name)s - %(levelname)s - %(message)s')
f_format = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
w_handler.setFormatter(c_format)
e_handler.setFormatter(f_format)
# Add handlers to the logger
logger_obj.addHandler(w_handler)
logger_obj.addHandler(e_handler)
# Generate log messages
logger_obj.warning('This is a warning message')
logger_obj.error('This is an error message')
- Understanding the Output: When this script is run, it generates two types of outputs based on the configured handlers:
- Console Output:
- Displays warnings, formatted as specified in c_format;
- Example: __main__ – WARNING – This is a warning message
- File Output:
- Records errors in a file, using the format defined in f_format;
- Example: 2023-03-15 10:00:00,000 – __main__ – ERROR – This is an error message
Deep Dive into Handler Mechanics:
In the provided example, the logger logger_obj creates a LogRecord for each logging event. This LogRecord is an essential component that encapsulates all the information related to a logging event.
- Stream Handler (w_handler):
- Set to capture and process log messages at or above the WARNING level;
- Formats the log message using c_format and outputs it to the console.
- File Handler (e_handler):
- Configured to handle only ERROR level messages;
- Utilizes f_format to format log messages and saves them in the specified log file.
Conclusion
The logging module is renowned for its unparalleled versatility, allowing you to monitor and display logging records while presenting users with tailored messages. Furthermore, it grants you the liberty to craft distinct handler classes, set custom log levels, and employ a plethora of invaluable techniques. We trust that this article has proven to be of great assistance to you.