Exception handling plays a pivotal yet often underappreciated role in the realm of programming languages, serving as an uncelebrated hero that silently guarantees the seamless functioning of your code, even when unexpected errors unexpectedly emerge. Within the domain of VB.Net, this crucial capability certainly does not go unnoticed. In this article, we will embark on an in-depth exploration of the universe of exceptions within VB.Net, with a primary focus on the adept and methodical utilization of try-catch blocks to expertly handle exceptions.
When a code stumbles upon an exception, there are two divergent paths that it can tread on:
Simply turn a blind eye to the exception, hoping it will disappear on its own. This, however, is not a recommended approach as neglecting exceptions can spawn unpredictable consequences.
Tackle the exception tactfully without disrupting the normal functioning of the code, which is the more advisable route.
Let’s now delve into the essence of exception handling.
Understanding Exception Handling in Detail
Error management plays a pivotal role in the realm of programming languages, encompassing the vital tasks of pinpointing, foreseeing, and taming programming irregularities or exceptions. This mechanism functions as a protective barrier, enabling the seamless execution of code even in the face of errors, consequently upholding the continuous performance of applications. Proficient utilization of exception handling carries the potential to substantially enhance the clarity, sustainability, and dependability of code. Undoubtedly, it stands as an indispensable asset within a developer’s arsenal, guaranteeing the creation of meticulously structured and robust applications.
Unlocking the Intricacies of Exception Handling in Programming
Exception handling serves as a crucial safety net in the realm of programming, a guardian angel that watches over your code, ready to step in when errors threaten to disrupt its smooth operation. This comprehensive guide will take you on a journey through the intricacies of exception handling, revealing its core elements, strategies, and best practices that can help you harness its power effectively.
The Essence of Exception Handling
Exception handling, at its core, is the art of managing errors that may rear their heads during the execution of a software application. Instead of allowing a program to crash abruptly when encountering errors, it provides a structured approach to handle these issues gracefully, ensuring the application continues to run smoothly.
Key Elements of Exception Handling
To understand exception handling fully, you must grasp its essential components:
- Identification and Anticipation of Errors: The first step is identifying potential error points within your code. This involves thorough analysis and a proactive approach to foresee issues;
- Effective Error Response Mechanisms: Exception handling is not just about spotting errors; it’s about responding to them effectively. You need mechanisms in place to catch these errors as they occur and take appropriate action;
- Ensuring Robustness: Exception handling aims to maintain program functionality and user-friendliness even in the face of unforeseen errors. This requires strategic planning and preparation.
Delving Deep into Try-Catch Blocks
Think of try-catch blocks as protective shields within your code, guarding it against potential pitfalls. They work in a straightforward manner:
- The ‘try’ Block: Code that might trigger exceptions is enclosed within a ‘try’ block, creating a protected zone where errors can be safely monitored;
- The ‘catch’ Block: When an exception occurs, the ‘catch’ block comes to the rescue. It contains code specifically designed to address the error and facilitate the program’s recovery.
Insights on Utilizing Try-Catch Blocks
Now, let’s dive into some practical insights for using try-catch blocks effectively:
- Strategic Placement: Critical code that is susceptible to failure under certain conditions should find its home within the ‘try’ block, ensuring that it’s under the protective umbrella of exception handling;
- Custom Error Handling: Within ‘catch’ blocks, you can define specific actions that should occur when an error is caught, tailoring your response to the nature of the error;
- Error Logging: Consider logging error details within the ‘catch’ block. This practice aids in future analysis and debugging, providing valuable insights into what went wrong and why.
Revisiting the Crucial Role of Exception Handling
Exception handling isn’t just a safety net; it’s a proactive approach to identify and manage potential code errors. Its heart lies in the strategic use of try-catch blocks, preventing program crashes, and offering clarity through step-by-step error messages.
Benefits of Effective Exception Handling
Embracing effective exception handling brings an array of benefits to your software development journey:
- Enhanced Reliability: Your software becomes more reliable and robust, capable of handling unexpected challenges without breaking down;
- User Trust and Confidence: Minimize disruptions due to errors, enhancing user trust and confidence in your application. Users are more likely to return to software that provides a smooth experience;
- Developer-Friendly Code: Developers will find it easier to maintain and debug the code when it’s fortified with a well-thought-out exception handling mechanism.
Recommendations for Robust Exception Handling
To truly master exception handling, consider these best practices:
- Continuous Improvement: Regularly update and refine your exception handling logic based on new insights and user feedback. The software environment is dynamic, and your error-handling strategy should evolve accordingly;
- Comprehensive Testing: Thoroughly test your code under various scenarios to ensure that all potential exceptions are adequately handled. This proactive approach can save you from unexpected headaches down the road;
- Meaningful Error Messages: Provide users with error messages that not only notify them of a problem but also guide them in understanding and, if possible, resolving the issue. Clear communication is key to a positive user experience.
Now that you have a solid understanding of the essence of exception handling and its key elements, let’s delve into its implementation in VB.Net, uncovering the specific techniques and practices that make it a powerful tool in this programming language.
Mastering Exception Handling in VB.Net: A Comprehensive Guide
Exception handling is not merely a technique; it is the bedrock of creating robust and reliable software applications in VB.Net. When done right, it acts as an invisible shield, protecting your code from unexpected errors and ensuring seamless user experiences. This comprehensive guide will take you on a journey through the intricate world of exception handling in VB.Net, from the basics to advanced techniques, best practices, and valuable tips.
Understanding the Core of Exception Handling in VB.Net
Exception handling in VB.Net is akin to being a vigilant guardian of your code, ready to tackle unexpected events or errors that might disrupt its flow. By embracing the Try-Catch-Finally framework, you’ll fortify your applications and ensure their uninterrupted operation.
Key Components of Exception Handling in VB.Net
- Try Block: The Front Line of Defense
- In the Try block, you encapsulate the code that might throw exceptions;
- This block is where your program attempts to execute potentially error-prone operations.
- Catch Block: The Problem Solver
- When an exception occurs, the Catch block springs into action;
- It contains the logic to manage the exception, whether that’s logging the error, providing user-friendly messages, or implementing a recovery strategy.
- Finally Block: The Cleanup Crew
- The Finally block is a vital resource management tool;
- It executes regardless of whether an exception was thrown or not, making it ideal for tasks like releasing resources or closing file connections.
- Throw Keyword: Raising the Flag
- The Throw keyword allows you to explicitly raise an exception;
- Whether you’re re-throwing a caught exception or creating a new one, it signals the presence of an issue that demands attention from a Catch block.
Advanced Techniques in Exception Handling
As you delve deeper into the world of VB.Net exception handling, you’ll encounter advanced strategies to finesse your error management:
1. Creating Custom Exceptions
Predefined exceptions are like standard tools, but sometimes, you need a specialized instrument to get the job done. This is where creating custom exceptions comes into play.
Why Custom Exceptions Matter
- They allow you to convey specific error conditions that predefined exceptions might miss;
- Custom exceptions make your code more expressive and precise;
- Tailor them to your application’s unique needs, enhancing clarity and debugging.
2. Proprietary Exception Classes
For applications with intricate error handling requirements, proprietary exception classes are a godsend. They go beyond the standard exceptions by including additional information:
Advantages of Proprietary Exception Classes
- You can embed error codes, contextual data, or even detailed descriptions within these classes.
- This data facilitates efficient debugging and error tracking;
- Your error messages become more informative and actionable.
Best Practices and Tips for Effective Exception Handling
Now that you’re well-versed in the fundamentals and advanced techniques, let’s explore some best practices and invaluable tips to elevate your exception handling game:
1. Use Specific Catch Blocks
Rather than casting a wide net with generic Catch blocks, target specific exceptions. This not only enhances error specificity but also makes your code more readable and maintainable.
2. Avoid Empty Catch Blocks
Empty Catch blocks are like dark alleys in your code, concealing problems and making debugging an uphill battle. Always include meaningful handling logic in your Catch blocks to shed light on issues.
3. Log Detailed Information
When an exception strikes, don’t skimp on the details. Log as much information as possible to create a breadcrumb trail leading to the root cause of the problem. This expedites diagnosis and resolution.
4. Use the Finally Block Wisely
The Finally block is a powerful tool, but wield it with care. Reserve it for essential cleanup tasks, like resource release. Misusing it can introduce further complications.
5. Avoid Overusing Throw
While the Throw keyword is a lifesaver, avoid overusing it. Reckless throwing can clutter your code and muddy its flow. Use it judiciously, deploying it only when necessary to signal critical issues.
Exception handling is an art as much as it is a science. By mastering it, you’ll become a more proficient VB.Net developer, capable of crafting software that not only works but also shines in the face of adversity.
Implementing Exception Handling: An Illustration
To illustrate how these keywords work in tandem, consider a block of code that might create an exception. We encapsulate this block within the ‘Try-Catch’ construct, turning it into what is known as ‘protected code’. Here’s the syntax involved:
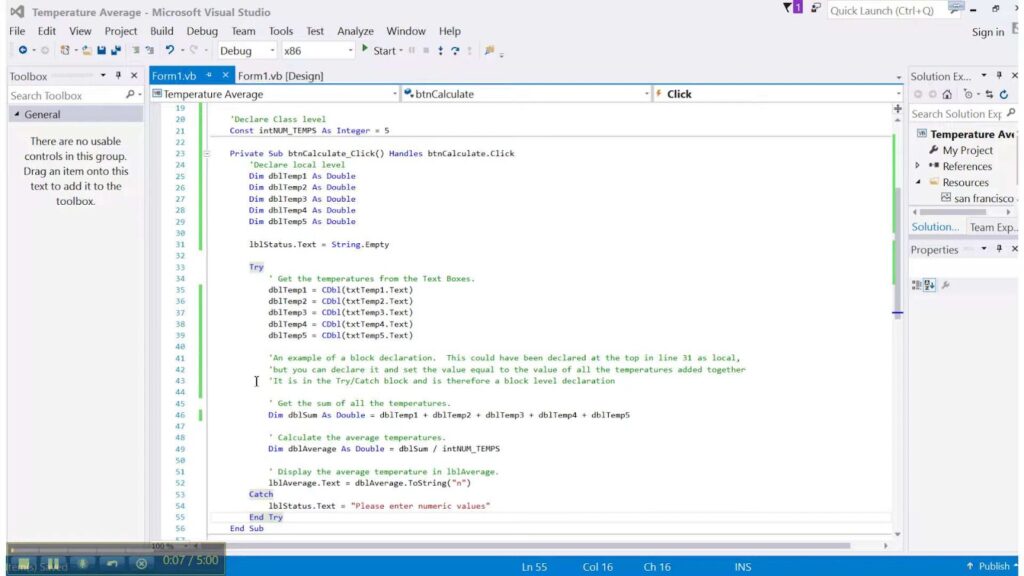
To tackle scenarios where multiple exceptions could be thrown, you can use multiple ‘Catch’ statements corresponding to each exception type.
Now, let’s delve into the system-provided System.ApplicationException.
Illustrating Exception Logging in VB.Net: Embracing the ClearInsights SDK
Given the crucial role of exception handling, enhancing it through systematic logging can be a game-changer. It provides pertinent insights into the issues arising in your application, allowing you to address problems accurately and promptly. The ClearInsights Logging library, well-known for its ease of integration and application, can be an excellent tool in your VB.Net exception handling arsenal.
Let’s dive into a hands-on example showcasing how to log exceptions using the ClearInsights SDK:
' Import the required libraries
Imports ClearInsights.Logging
Imports Microsoft.Extensions.DependencyInjection
Imports Microsoft.Extensions.Logging
Module Program
Sub Main(args As String())
' Create service collection to register services
Dim serviceCollection As New ServiceCollection()
' Call method to configure services and add logging
ConfigureServices(serviceCollection)
' Build the service provider
Dim serviceProvider = serviceCollection.BuildServiceProvider()
' This will automatically catch any unhandled exceptions
System.AppDomain.CurrentDomain.UseClearInsightsExceptionHandling(serviceProvider.GetService(Of ILogger(Of AppDomain)))
' Get an instance of the class with the code to execute
Dim someClassInstance = serviceProvider.GetService(Of SomeClass)()
' Execute methods with exception handling
someClassInstance.ExecuteHandledException()
someClassInstance.ExecuteUnHandledException()
End Sub
Private Sub ConfigureServices(ByVal services As IServiceCollection)
services.AddLogging(Function(configure)
Return configure.AddClearInsightsLogger(Sub(configuration)
configuration.ApiKey = "{Your ApiKey}"
configuration.Secret = "{Your Secret}"
configuration.ApplicationName = "{Your Application Name}"
End Sub)
End Function).AddTransient(Of SomeClass)()
End Sub
End Module
Public Class SomeClass
Private ReadOnly _logger As ILogger(Of SomeClass)
Public Sub New(logger As ILogger(Of SomeClass))
_logger = logger
End Sub
Public Sub ExecuteHandledException()
Try
Throw New ArgumentNullException()
Catch ex As Exception
_logger.LogError(ex, ex.Message)
End Try
End Sub
Public Sub ExecuteUnHandledException()
Throw New ArgumentNullException()
End Sub
End Class
In this example, the ‘SomeClass’ class houses two methods – ExecuteHandledException() and ExecuteUnHandledException(). Both methods throw an ArgumentNullException, but the ExecuteHandledException() method logs the exception using the ILogger instance.
The ConfigureServices method integrates the ClearInsights logger with the .NET Core logging infrastructure. It creates the service collection, configures the logger with the appropriate API key, secret, and Application name, and finally registers the ‘SomeClass’ class with transient lifetime.
This example underlines the significance of logging in enhancing exception handling, providing crucial insights into your applications, and allowing you to address issues efficiently. Also, Dive into the art of C# exception handling and become a coding maestro! Learn how to conquer errors with finesse in this insightful guide.
Wrapping Up: The Power of Exception Handling in VB.Net
In the world of software development, it’s not a question of if your code will encounter errors, but when. Resilient code can weather these unexpected storms, and that’s where exception handling comes into play. Exception handling, especially in a robust language like VB.Net, equips developers with powerful tools to stand up to these unexpected issues, preventing your software from coming to an unexpected halt.
Throughout this article, we delved into the crux of exception handling in VB.Net, exploring elements such as ‘Try’, ‘Catch’, ‘Finally’ blocks and more. We also looked at how the .NET framework classifies exceptions and investigated the practical implementation of exception handling in VB.Net.
Armed with this knowledge, you—the developer—are now better equipped to craft resilient, high-performing code. Not only can you prevent potentially catastrophic crashes, but you can also improve the overall user experience by preventing unexpected interruptions.
In conclusion, no matter what programming language you are using, remember that a key trait of a good programmer is the ability to anticipate, prepare for, and gracefully manage the unexpected. So keep coding, stay curious, and always strive to improve your understanding and handling of exceptions.