In the world of software development, encountering errors and issues is an inevitable part of the journey. One such error that often leaves developers scratching their heads is the infamous “Unable to resolve service for type Microsoft.Extensions.Logging.ILogger” error. This error message can be a roadblock when working with .NET Core or ASP.NET Core applications, causing frustration and delaying project progress.
However, fret not, as this article aims to shed light on this common error and guide you through the process of solving it effectively. We’ll dive into what the error means, why it occurs, and, most importantly, how to fix it. By the end of this article, you’ll have a clear understanding of the root causes behind this error and the necessary steps to resolve it, ensuring a smoother and more efficient development experience with Microsoft’s powerful logging framework.
Recognizing the Problem: ‘Service Type Resolution Failure’
In the process of deploying .Net applications that make use of the Microsoft ILogger class, you may encounter a runtime issue. This particular error is known as System.InvalidOperationException, and it presents a message that reads as follows: ‘Service type resolution failure for ‘Microsoft.Extensions.Logging.ILogger”. This situation frequently arises, particularly when the dependencies within the ILogger interface have not been properly configured.
A Step-by-Step Guide to Replicate this Issue
This error is fairly common and can be replicated following certain configurations.
Configuration 1 — Configuring Main Console Application
Here is a typical console application Main configuration that could potentially lead to the error:
var services = new ServiceCollection()
.AddLogging(logging => logging.AddConsole())
.BuildServiceProvider();
In this configuration, a logging service is added to the ServiceCollection and then built into a service provider.
Class Implementation
Consider a class implementation like this one:
private readonly ILogger _logger;
public MyService(ILogger logger)
{
_logger = logger;
}
public void Execute()
{
_logger.LogInformation("My Message");
}
The program has been meticulously crafted to record data by employing the instance _logger derived from ILogger. This valuable instance derives its value from the logger provided during the construction of MyService. Nevertheless, in the event of an erroneous arrangement of dependencies, the program may stumble upon a predicament, unable to ascertain the ILogger type when constructing MyService, thereby resulting in the previously mentioned error.
Key Points to Keep in Mind
To avert such predicaments, it becomes imperative to:
- Thoroughly validate the accurate configuration of dependency injection;
- Assure the impeccable implementation of the Microsoft ILogger class;
- Verify the precise setup of both the console application’s Main function and the class implementation.
The Resolution: Correct Application of ILogger
The error ‘Unable to resolve service for type ‘Microsoft.Extensions.Logging.ILogger’’ is commonly a result of incorrect ILogger registration. Understanding how to apply the appropriate ILogger variant can resolve this issue.
Configuration Correction
The main shift is from a non-generic ILogger to a generic one, i.e., ILogger<T>. The latter is automatically registered in .NET applications, unlike the former. Here’s a comparison to illustrate this:
private readonly ILogger _logger;
public MyService(ILogger logger)
{
_logger = logger;
}
public void Execute()
{
_logger.LogInformation("My Message");
}
In the above example, a non-generic ILogger is improperly registered, leading to the error.
Correct Registration of ILogger
private readonly ILogger _logger;
public MyService(ILogger<MyService> logger)
{
_logger = logger;
}
public void Execute()
{
_logger.LogInformation("My Message");
}
In this example, the ILogger<T> variant is appropriately registered with the class MyService, thereby preventing the error.
ILogger Usage in Startup.cs or Main.cs
You may also need to use the ILogger in the Startup.cs or Main.cs classes. Here’s how:
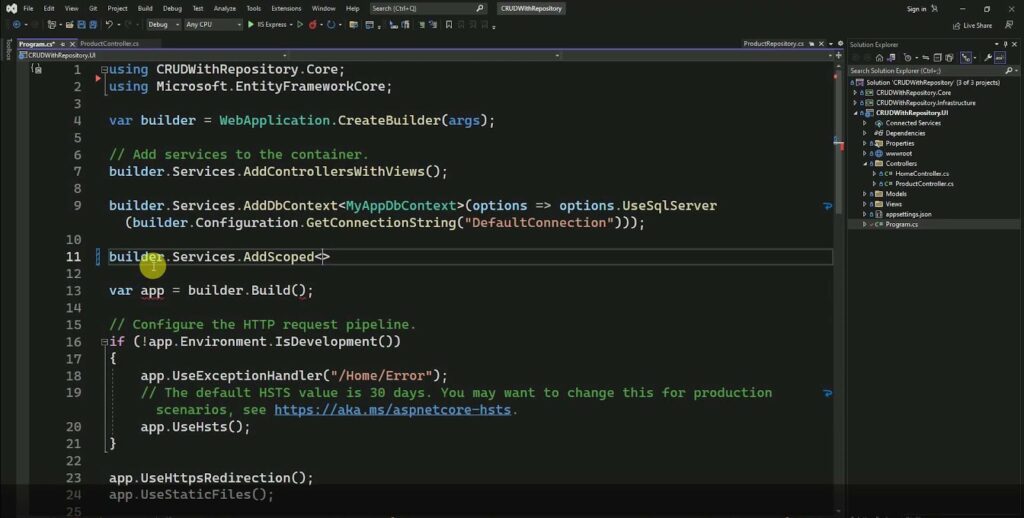
Create a Generic Class
For ILogger<T>, ‘T’ represents a category that you need to define. In this case, a generic class named ‘ApplicationLogger’ is created:
public class ApplicationLogger
{
}
Use ILogger with Generic Class in Startup or Main
This step involves injecting the instance of ILogger<ApplicationLogger> to the service provider. If you plan to use the logger across the application, register it with Singleton to ensure a single logger instance throughout the application.
public void ConfigureServices(IServiceCollection services)
{
var serviceProvider = services.BuildServiceProvider();
var logger = serviceProvider.GetService<ILogger<ApplicationLogger>>();
services.AddSingleton(typeof(ILogger), logger);
}
This setup addresses the ‘Unable to resolve service for type’ error, ensuring your .NET applications run smoothly. Also, Discover the art of preserving Python’s secrets on file! Explore best practices for efficient Python logging to files in this comprehensive guide.
Conclusion
To sum up, encountering the error “Unable to resolve service for type ‘Microsoft.Extensions.Logging.ILogger'” can initially seem daunting for developers. However, with appropriate strategies and thorough troubleshooting, this hurdle is certainly surmountable. This discussion delved into a spectrum of potential factors that might trigger this error, including absent dependencies, configuration inaccuracies, and complications related to the dependency injection container. By meticulously examining the error details, verifying configurations for accuracy, and confirming the proper registration of all necessary services and dependencies, developers are well-equipped to address this problem, ensuring the seamless operation of their applications.